Create People Picker using SharePoint Hosted App
Here, I demonstrated how to create a People picker using Javascript
Example code for JSOM:
1.Open Default.aspx page.
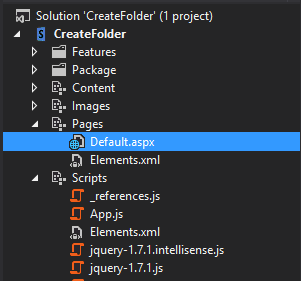
Add below code
2. Open App.js file and add below code
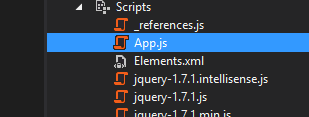
Example code for JSOM:
1.Open Default.aspx page.
Add below code
<%-- The markup and script in the following Content element will be placed in the <body> of the page --%> <asp:Content ContentPlaceHolderID="PlaceHolderMain" runat="server"> <SharePoint:ScriptLink ID="ScriptLink1" name="clienttemplates.js" runat="server" LoadAfterUI="true" Localizable="false" /> <SharePoint:ScriptLink ID="ScriptLink2" name="clientforms.js" runat="server" LoadAfterUI="true" Localizable="false" /> <SharePoint:ScriptLink ID="ScriptLink3" name="clientpeoplepicker.js" runat="server" LoadAfterUI="true" Localizable="false" /> <SharePoint:ScriptLink ID="ScriptLink4" name="autofill.js" runat="server" LoadAfterUI="true" Localizable="false" /> <SharePoint:ScriptLink ID="ScriptLink5" name="sp.js" runat="server" LoadAfterUI="true" Localizable="false" /> <SharePoint:ScriptLink ID="ScriptLink6" name="sp.runtime.js" runat="server" LoadAfterUI="true" Localizable="false" /> <SharePoint:ScriptLink ID="ScriptLink7" name="sp.core.js" runat="server" LoadAfterUI="true" Localizable="false" /> <div id="peoplePickerDiv"></div> <div> <br/> <input type="button" value="Get User Info" onclick="getUserInfo()"></input> <br/> <h1>User info:</h1> <p id="resolvedUsers"></p> <h1>User keys:</h1> <p id="userKeys"></p> <h1>User ID:</h1> <p id="userId"></p> </div>
</asp:Content>
2. Open App.js file and add below code
'use strict'; var context = SP.ClientContext.get_current(); var user = context.get_web().get_currentUser(); // This code runs when the DOM is ready and creates a context object which is needed to use the SharePoint object model $(document).ready(function () { // Specify the unique ID of the DOM element where the // picker will render. SP.SOD.executeFunc('clientpeoplepicker.js', 'SPClientPeoplePicker', function () { initializePeoplePicker('peoplePickerDiv'); }); }); // Render and initialize the client-side People Picker. function initializePeoplePicker(peoplePickerElementId) { // Create a schema to store picker properties, and set the properties. var schema = {}; schema['PrincipalAccountType'] = 'User,DL,SecGroup,SPGroup'; schema['SearchPrincipalSource'] = 15; schema['ResolvePrincipalSource'] = 15; schema['AllowMultipleValues'] = true; schema['MaximumEntitySuggestions'] = 50; schema['Width'] = '280px'; // Render and initialize the picker. // Pass the ID of the DOM element that contains the picker, an array of initial // PickerEntity objects to set the picker value, and a schema that defines // picker properties. SPClientPeoplePicker_InitStandaloneControlWrapper(peoplePickerElementId, null, schema); } // Query the picker for user information. function getUserInfo() { // Get the people picker object from the page. var peoplePicker = this.SPClientPeoplePicker.SPClientPeoplePickerDict.peoplePickerDiv_TopSpan; // Get information about all users. var users = peoplePicker.GetAllUserInfo(); var userInfo = ''; for (var i = 0; i < users.length; i++) { var user = users[i]; for (var userProperty in user) { userInfo += userProperty + ': ' + user[userProperty] + '<br>'; } } $('#resolvedUsers').html(userInfo); // Get user keys. var keys = peoplePicker.GetAllUserKeys(); $('#userKeys').html(keys); // Get the first user's ID by using the login name. getUserId(users[0].Key); } // Get the user ID. function getUserId(loginName) { var context = new SP.ClientContext.get_current(); this.user = context.get_web().ensureUser(loginName); context.load(this.user); context.executeQueryAsync( Function.createDelegate(null, ensureUserSuccess), Function.createDelegate(null, onFail) ); } function ensureUserSuccess() { $('#userId').html(this.user.get_id()); } function onFail(sender, args) { alert('Query failed. Error: ' + args.get_message()); }
Comments
Post a Comment